CIS 260 - Graphical TicTacToe (GUI)
The objectives of this assignment are:
- create a GUI to play TicTacToe
- use your previously implemented TitTacToe class
Assignment
This assignment is all about creating and using a Java GUI and reusing
a class you have previously designed. You should already have a TicTacToe
class which represents a TicTacToe board. You now have to create a GUI
interface to replace the text interface you previously used.
Requirements for your Java class
- Make sure your old TicTacToe.java code is in the same directory.
- It should extend a JFrame and implement the MouseListener interface.
- It should contain a private class named TTTLabel. TTTLabel should
extend JLabel and add a public instance integer field named
tag. The field should be initialized to -1.
- It should contain the following two fields:
- an array of nine TTTLabel objects
- a reference to a TicTacToe object
- It should contain eight methods
- main(String[]): create a new object of itself
- a constructor which will call createAndShowGUI when the
event dispatch thread is ready to run it; it should send
a title string ("Tic-Tac-Toe") to createAndShowGUI
- createAndShowGUI(String): see notes below for
detailed requirements
- mouseClicked(MouseEvent): see notes below for
detailed requirements
- mouseEntered(MouseEvent): do nothing
- mouseExited(MouseEvent): do nothing
- mousePressed(MouseEvent): do nothing
- mouseReleased(MouseEvent): do nothing
- createAndShowGUI(String) should do the following:
- create a new TicTacToe object
- set the layout of the content pane of the object we are
in to be a GridLayout
- create nine TTTLabel objects to populate the array
of TTTLabels, set the tags for those labels to
the numbers 1 through 9, set the text to a blank,
add the current object as a mouse listener to each
label, set the borders and styling on each cell to
make it look like the example, and add each cell to
the content pane of the object we are in
- set the default close operation
- make the object we are in 300 pixels wide and 300 pixels
tall, and make it visible
- mouseClicked(MouseEvent) should do the following:
- get the tag value from the label that was clicked
- find out from the TicTacToe object what player's move it is
- use the tag value and the TicTacToe object to try to make
the move that was requested by the mouse click
- if the move was valid/accepted, then set the text in the
label that was clicked to the correct player character,
check the status of the TicTacToe object, and display an
appropriate message if the game is no longer
TicTacToe.Status.ONGOING
- if the game is no longer ongoing, then exit
Sample
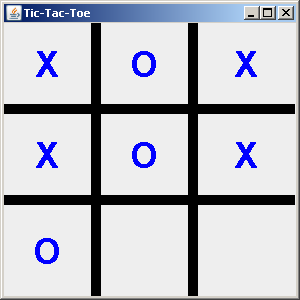
Grading rubric
Note: Program must compile without errors.
- 5 points for following style conventions (no tabs, proper indentation, documentation comments)
- 35 points for the GUI working properly
- 10 points for the game working properly